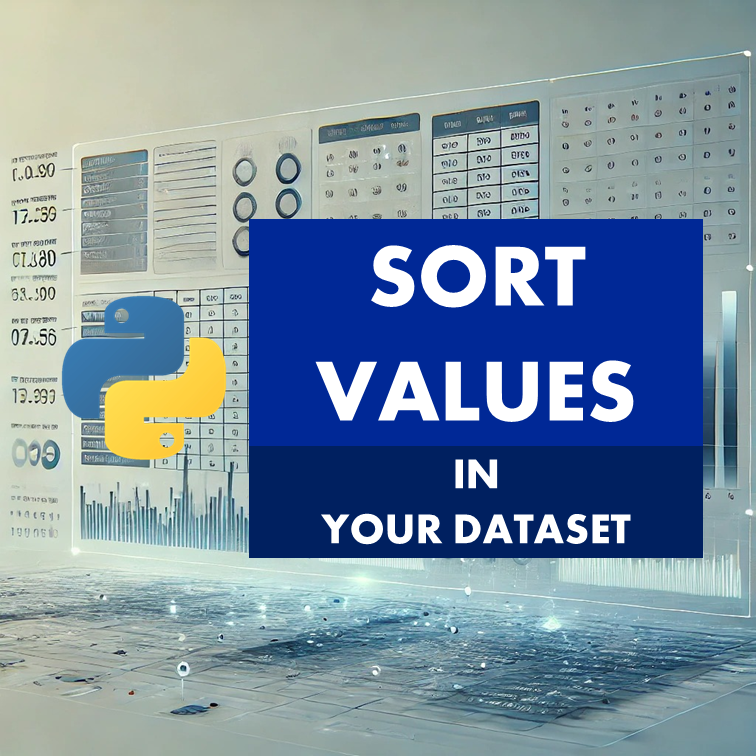
Welcome back to PyGinners! In this blog post, we’ll explore the sort_values
method in Python, a powerful tool for sorting data. Whether you’re new to Python or just getting started with data analysis, this guide will walk you through each step.
About the Sorting Method in Python
The sort_values
method in Pandas is a powerful tool for organizing data. It allows you to sort a DataFrame based on the values of one or more columns. This method is particularly useful for ranking data, identifying trends, and making your data easier to interpret.
Dataset
The dataset used in this article, ‘US_Olympics.csv’, contains information about the United States’ participation in the Olympics. It includes the number of male and female athletes in various sports, as well as the total number of athletes in each sport. This data provides a comprehensive view of the US Olympic team composition, making it ideal for practicing data analysis techniques. You can download it here.
Getting Started
Before we dive into the code, make sure you have Pandas installed. If you haven’t installed it yet, you can do so using pip:
pip install pandas
What is Pandas?
Pandas is a popular Python library for data manipulation and analysis. It provides data structures and functions needed to work efficiently with structured data, like tables. Pandas is essential for data analysis tasks because it allows you to load, prepare, manipulate, model, and analyze large amounts of data with ease.
Importing Pandas and Loading Data
First, we need to import the Pandas library and load our dataset. For this example, we’ll be using a CSV file named ‘US_Olympics.csv’. This dataset contains information about the United States’ participation in the Olympics.
# Import the pandas library
import pandas as pd
# Load the dataset into a DataFrame
df = pd.read_csv('US_Olympics.csv')
Inspecting the First Few Rows
To get a quick look at the data, we can use the head
method, which displays the first five rows of the DataFrame by default. This helps us understand the structure and contents of the dataset.
# Display the DataFrame
print(df)
The output will of the dataset looks like this:
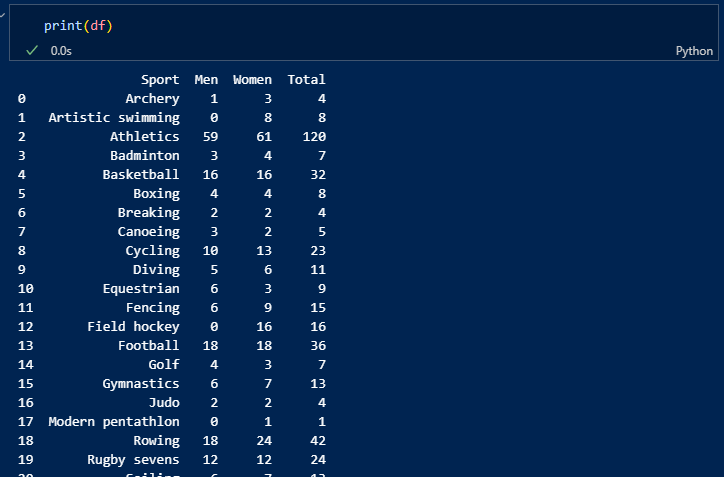
Sorting the Data
Now, let’s explore the sort_values
method. This method sorts the DataFrame based on the values of a specific column.
Sorting by Total Athletes
To sort the DataFrame by the ‘Total’ column in descending order, you can use the following code:
# Sort the DataFrame by the 'Total' column in descending order
df_sorted = df.sort_values('Total', ascending=False)
This will return a DataFrame sorted by the ‘Total’ column, with the highest values first.
Understanding the Output
The sorted DataFrame will look like this:
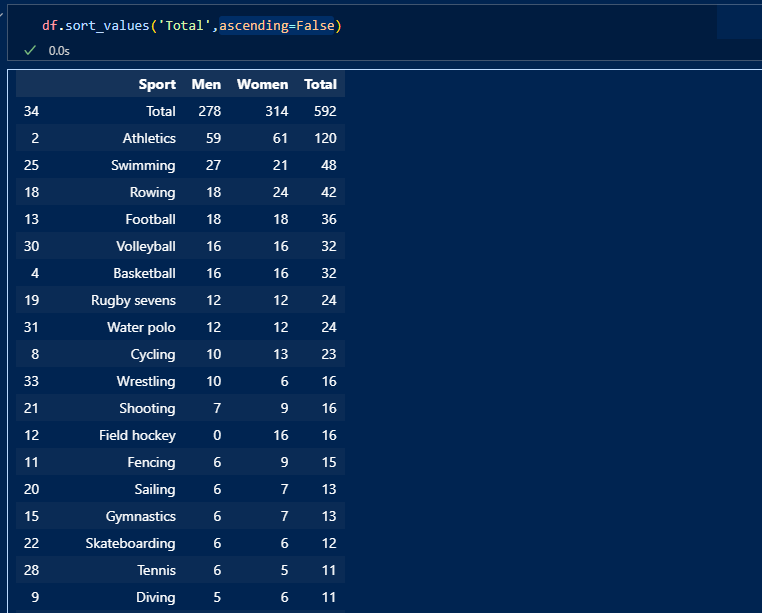
In this output, the rows are ordered by the ‘Total’ column, starting with the highest total number of athletes. By default the values are in ascending order, which is why we have to set the parameter to ascending=False for our purposes.
Putting It All Together
Here’s the complete code with comments explaining each step:
Import the pandas library
import pandas as pd
# Load the dataset into a DataFrame
df = pd.read_csv('US_Olympics.csv')
# Display the first five rows of the DataFrame to understand its structure
df.head()
# Sort the DataFrame by the 'Total' column in descending order
df_sorted = df.sort_values('Total', ascending=False)
Conclusion
The sort_values
method in Pandas is a powerful tool for sorting your data. By following this guide, you should now have a good understanding of how to use the sort_values
method in Pandas. Try it out with your own data and see how it can help you organize and analyze your datasets more effectively.
Stay tuned for more beginner-friendly tutorials on PyGinners.