
In this blog post, we’re going to explore the sample
method in Pandas. This method is incredibly useful when you want to randomly select rows or columns from your dataset. Whether you’re new to Python or data analysis, don’t worry! We’ll walk you through each step, ensuring you understand how to use this method effectively.
The Dataset
This dataset contains information about saltwater fishing sites in New York City. You can download it here.
Getting Started
Before we dive into the code, make sure you have Pandas installed. Pandas is a powerful and widely-used Python library for data manipulation and analysis. It provides data structures and functions needed to work seamlessly with structured data, such as tables (think Excel spreadsheets). Pandas is essential for data analysis tasks because it allows you to load, prepare, manipulate, model, and analyze large amounts of data efficiently.
If you haven’t installed it yet, you can do so using pip:
pip install pandas
Importing Pandas and Loading Data
First, we need to import the Pandas library and load our dataset. For this example, we’ll be using a CSV file named ‘NYC_Saltwater_Fishing_Sites.csv’.
Here’s how you can do it:
# Import the pandas library
import pandas as pd
# Load the dataset into a DataFrame
df = pd.read_csv('NYC_Saltwater_Fishing_Sites.csv')
Inspecting the First Few Rows
To get a quick look at the data, we can use the head
method, which displays the first five rows of the DataFrame by default. This helps us understand the structure and contents of the dataset.
# Display the first five rows of the DataFrame
df.head()

Random Sampling with sample
Now, let’s explore the sample
method. This method allows us to randomly select a specified number of rows or columns from the DataFrame.
Randomly Sample One Row
If you want to randomly select one row from the DataFrame, you can use the sample
method without any arguments:
# Randomly select one row from the DataFrame
df.sample()
This will return a single row chosen at random from the DataFrame.
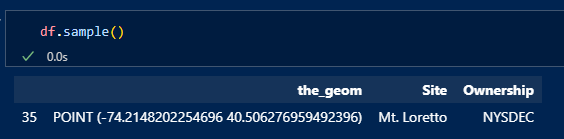
Randomly Sample Multiple Rows
To randomly select multiple rows, you can pass an integer as an argument to the sample
method. For example, to select seven random rows, you would use:
# Randomly select seven rows from the DataFrame
df.sample(7)
This will return seven rows chosen at random from the DataFrame.
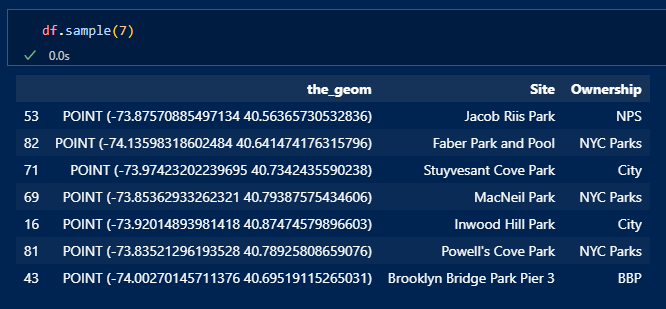
Putting It All Together
Here’s the complete code with comments explaining each step:
# Import the pandas library
import pandas as pd
# Load the dataset into a DataFrame
df = pd.read_csv('NYC_Saltwater_Fishing_Sites.csv')
# Display the first five rows of the DataFrame to understand its structure
df.head()
# Randomly select one row from the DataFrame
df.sample()
# Randomly select seven rows from the DataFrame
df.sample(7)
Conclusion
The sample
method in Pandas is a powerful tool for data analysis, allowing you to randomly select rows or columns from your dataset. This can be particularly useful for testing, creating smaller datasets for exploration, or performing statistical analysis.
By following this guide, you should now have a good understanding of how to use the sample
method in Pandas. Try it out with your own data and see how it can help you in your data analysis tasks!
Stay tuned for more beginner-friendly tutorials on PyGinners. Happy coding!